Notice
Recent Posts
Recent Comments
Link
반응형
250x250
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
Tags
- mybatis
- HTML
- 웹앱
- 리액트세팅
- 구글 oauth
- CSS
- 리액트초기세팅
- js
- 자바스크립트 기초
- springboot
- 코딩
- Javascript
- 웹
- Spring Boot
- java
- javaspring
- 구글캘린더api
- 자바스크립트
- 마이바티스
- 기초코딩
- spring
- 처음만나는자바스크립트
- 스프링부트
- 전자정부 서버세팅
- 기초 코딩
- 자바스크립트기초
- react
- 자바스크립트기초문법
- 자바
- 리액트프로젝트세팅
Archives
- Today
- Total
인생 디벨로퍼
Ajax를 이용한 update (개인정보 수정) 본문
728x90
반응형
개인정보 수정 페이지에서,
ajax 를 이용한 put 요청을 해보쟈!
Controller
@Controller
public class EmployeeController {
@Autowired
private EmployeeRepository employeeRepository;
@Autowired
private EmployeeService employeeService;
@Autowired
private SchoolMasterRepository schoolMasterRepository;
@Autowired
private LicenseMasterRepository licenseMasterRepository;
@Autowired
private StackMasterRepository stackMasterRepository;
@Autowired
HttpSession session;
@PutMapping("/employee/{id}")
public ResponseEntity<?> update(@PathVariable int id, @RequestBody EmployeeUpdatdReq employeeUpdateReq) {
Employee emp = new Employee();
emp.setId(1);
emp.setEmployeeName("ssar");
emp.setEmployeePassword("1234");
session.setAttribute("principal", emp);
Employee principal = (Employee) session.getAttribute("principal");
if (principal == null) {
throw new CustomApiException("인증이 되지 않았습니다", HttpStatus.UNAUTHORIZED);
}
if (employeeUpdateReq.getEmployeePassword() == null ||
employeeUpdateReq.getEmployeePassword().isEmpty()) {
throw new CustomApiException("Password을 작성해주세요");
}
if (employeeUpdateReq.getEmployeeEmail() == null ||
employeeUpdateReq.getEmployeeEmail().isEmpty()) {
throw new CustomApiException("Email을 작성해주세요");
}
if (employeeUpdateReq.getEmployeeBirth() == null) {
throw new CustomApiException("Birth을 작성해주세요");
}
if (employeeUpdateReq.getEmployeeTel() == null ||
employeeUpdateReq.getEmployeeTel().isEmpty()) {
throw new CustomApiException("Tel을 작성해주세요");
}
if (employeeUpdateReq.getEmployeeAddress() == null ||
employeeUpdateReq.getEmployeeAddress().isEmpty()) {
throw new CustomApiException("Address을 작성해주세요");
}
employeeService.회원정보수정(employeeUpdateReq, principal.getId());
return new ResponseEntity<>(new ResponseDto<>(1, "회원정보 수정 완료!", null), HttpStatus.OK);
}
}
view (ajax)
<div class="my_yellow_box">
<div class="d-flex justify-content-between my_info_update_margin">
<div>
<div class="mb-2 d-flex align-items-center">
<span class="my_info_update_span">아이디</span>
<input class="form-control my_info_update_input" type="text" placeholder="Enter username" value="${empInfo.employeeName}" readonly/>
</div>
<div class="mb-2 d-flex align-items-center">
<span class="my_info_update_span">비밀번호</span>
<input class="form-control my_info_update_input" type="password" value="${empInfo.employeePassword}" id="employeePassword"/>
</div>
<div class="mb-2 d-flex align-items-center">
<span class="my_info_update_span">이메일</span>
<input class="form-control my_info_update_input" type="email" value="${empInfo.employeeEmail}" id="employeeEmail"/>
</div>
<div class="mb-2 d-flex align-items-center">
<span class="my_info_update_span">생년월일</span>
<input class="form-control my_info_update_input" type="date" value="${empInfo.employeeBirth}" id="employeeBirth"/>
</div>
<div class="mb-2 d-flex align-items-center">
<span class="my_info_update_span">연락처</span>
<input class="form-control my_info_update_input" type="text" value="${empInfo.employeeTel}" id="employeeTel"/>
</div>
<div class="d-flex align-items-center">
<span class="my_info_update_span">주소</span>
<input class="form-control my_info_update_input" type="text" value="${empInfo.employeeAddress}" id="employeeAddress"/>
</div>
</div>
<div class="d-flex align-items-center">
<div class="my_info_thumbnail me-4">
<img class="card-img-top" src="images/profile.jfif" alt="Card image"/>
</div>
</div>
</div>
</div>
<div class="button_center">
<a href=""></a>
<a onClick="updateById(${empInfo.id})" class="main_blue_btn" type="submit">수정</a>
</div>
<script>
function updateById(id) {
let data = {
employeePassword: $("#employeePassword").val(),
employeeEmail: $("#employeeEmail").val(),
employeeBirth: $("#employeeBirth").val(),
employeeTel: $("#employeeTel").val(),
employeeAddress: $("#employeeAddress").val()
};
console.log(data.employeeBirth);
$.ajax({
type: "put",
url: "/employee/" + id,
data: JSON.stringify(data),
contentType: "application/json; charset=utf-8",
dataType: "json" // default : 응답의 mime 타입으로 유추함
}).done((res) => { // 20X 일때
alert(res.msg);
location.href = "/employee/" + id + "/updateForm";
}).fail((err) => { // 40X, 50X 일때
alert(err.responseJSON.msg);
});
}
https://steponecoding.tistory.com/3
data 타입 날짜 받기
날짜 정보를 date 타입으로 받는다 날짜전송 @Controller public class HomeController { @GetMapping("/home") public String home() { return "home"; } } controller 와 view 를 간단히 만들어 테스트 한다. 1. "get" 데이터 형상을
steponecoding.tistory.com
date 타입을 처음 사용해 보며, 약간의 테스트가 필요했다
.xml (update 쿼리)
<update id="updateById">
update employee
set employee_password = #{employeeUpdatdReq.employeePassword}
,employee_email = #{employeeUpdatdReq.employeeEmail}
,employee_birth = #{employeeUpdatdReq.employeeBirth}
,employee_tel = #{employeeUpdatdReq.employeeTel}
,employee_address= #{employeeUpdatdReq.employeeAddress} where id = #{id}
</update>
Repository
public int updateById(@Param("id") int id, @Param("employeeUpdatdReq") EmployeeUpdatdReq employeeUpdatdReq);
DTO
@Getter
@Setter
public static class EmployeeUpdatdReq {
private String employeePassword;
private String employeeEmail;
private Date employeeBirth;
private String employeeTel;
private String employeeAddress;
}
Test
package shop.mtcoding.rodongin.controller;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.put;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
import java.sql.Date;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.boot.test.context.SpringBootTest.WebEnvironment;
import org.springframework.http.MediaType;
import org.springframework.mock.web.MockHttpSession;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.transaction.annotation.Transactional;
import com.fasterxml.jackson.databind.ObjectMapper;
import shop.mtcoding.rodongin.dto.employee.EmployeeReq.EmployeeUpdatdReq;
import shop.mtcoding.rodongin.model.employee.Employee;
@Transactional // 메서드 실행 직후 롤백!! // auto_increment 초기화
@AutoConfigureMockMvc
@SpringBootTest(webEnvironment = WebEnvironment.MOCK)
public class EmployeeControllerTest {
@Autowired
private MockMvc mvc;
@Autowired
private ObjectMapper om;
private MockHttpSession mockSession;
@BeforeEach
public void setUp() {
// 세션 주입
Employee employee = new Employee();
employee.setId(1);
employee.setEmployeeName("ssar");
employee.setEmployeePassword("1234");
employee.setEmployeeEmail("ssar@nate.com");
// employee.setEmployeeBirth(date);
employee.setEmployeeTel("01011111111");
employee.setEmployeeAddress("서울특별시 강남구");
mockSession = new MockHttpSession();
mockSession.setAttribute("principal", employee);
}
@Test
public void update_test() throws Exception {
// given
Date date = new Date(1990 - 01 - 12);
int id = 1;
EmployeeUpdatdReq employeeUpdatdReq = new EmployeeUpdatdReq();
employeeUpdatdReq.setEmployeePassword("1234");
employeeUpdatdReq.setEmployeeEmail("ssar@nate.com");
employeeUpdatdReq.setEmployeeBirth(date);
employeeUpdatdReq.setEmployeeTel("01022222222");
employeeUpdatdReq.setEmployeeAddress("부산 진구 부전동");
String requestBody = om.writeValueAsString(employeeUpdatdReq);
System.out.println("테스트 : " + requestBody);
// when
ResultActions resultActions = mvc.perform(
put("/employee/" + id)
.content(requestBody)
.contentType(MediaType.APPLICATION_JSON_VALUE)
.session(mockSession));
// then
resultActions.andExpect(status().isOk());
// resultActions.andExpect(jsonPath("$.code").value(1));
}
}
@BeforeEach 를 사용해, 세션의 주입
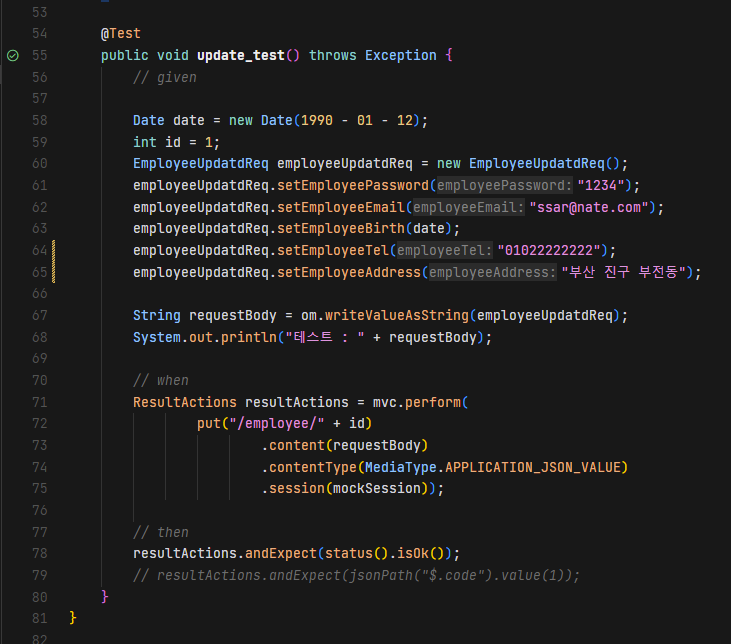

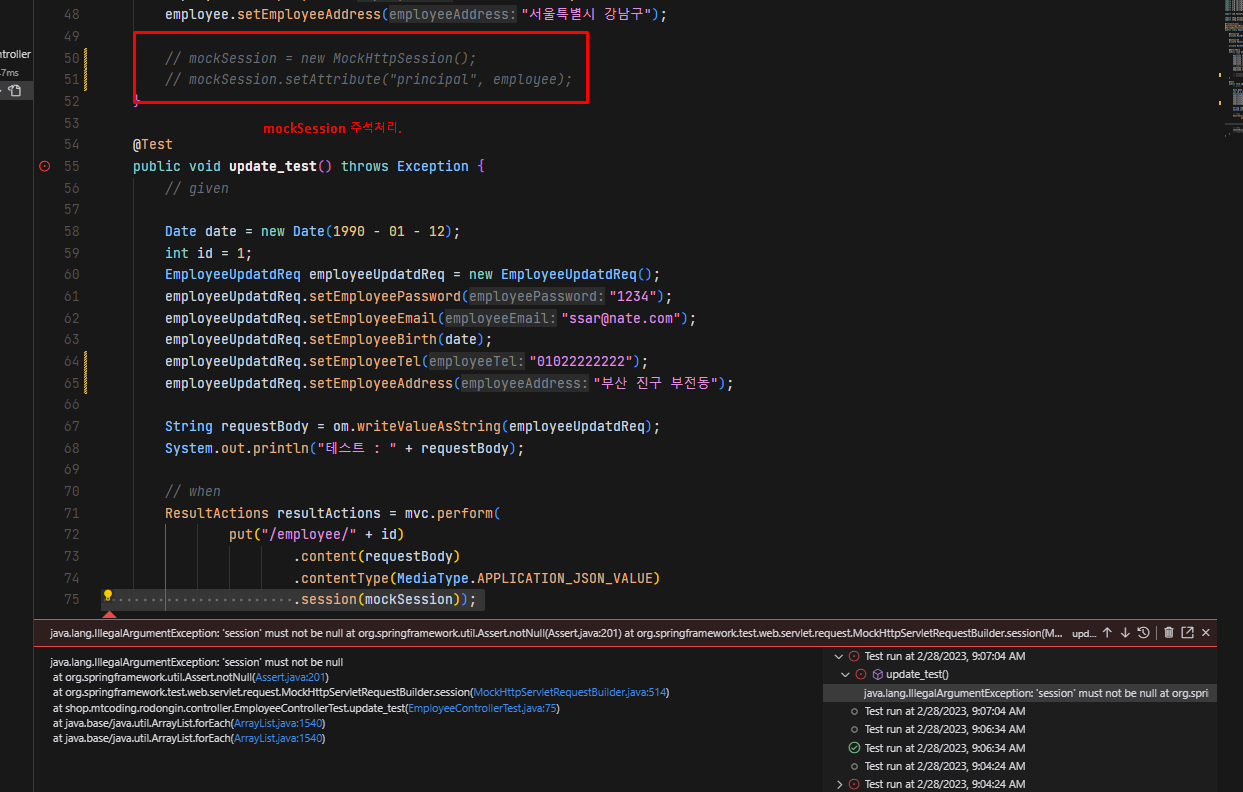
728x90
반응형
'Project > Mini Project - Rodonin (구인구직)' 카테고리의 다른 글
이력서 리스트 만들기 쿼리 / 절대 간단하지 않은 join (0) | 2023.03.07 |
---|---|
개인정보 뿌리기 (select, inner join) / 가장 간단한 inner join (0) | 2023.03.06 |
개인정보 detail 필수값 뿌리기 (select) (0) | 2023.03.06 |
개인정보 insert (form) (0) | 2023.03.06 |
date 타입 날짜 받기 (0) | 2023.02.27 |